Google reCaptcha is a free service from Google that helps protect websites from spam and abuse. Google has launched reCAPTCHA v3 to prevent spam bots without any user interaction. It returns us spam scores that can be used to take various actions in your web app.
What do I need to add the Google reCAPTCHA v3?
You only need a Google account to register your site to get API to use in your web application. So simple.
How do I get API Keys (Site Key and Secret Key)?
Please go to the official site of Google Captcha which is as below and click on “Admin Console”
https://www.google.com/recaptcha/intro/v3.html
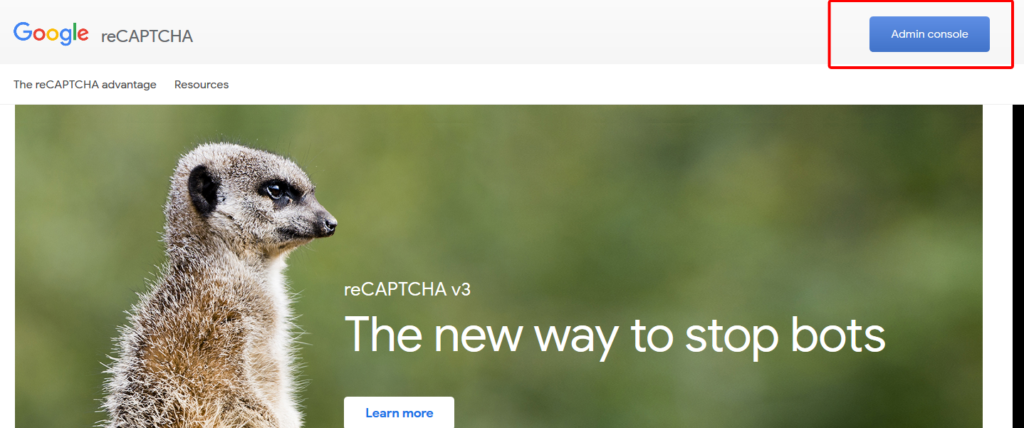
Once you click on “Admin Console”, it will ask you for your login details. Here you have to log in with your Google account.
Once you are logged-in, you will find a plus icon “+” on the top right side to “Create” and register a new website.
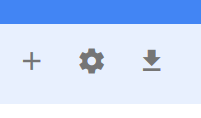
On the next screen, it’ll ask you for the details of your website to registered and where you wish to add Google reCaptcha. It will look like the below image.
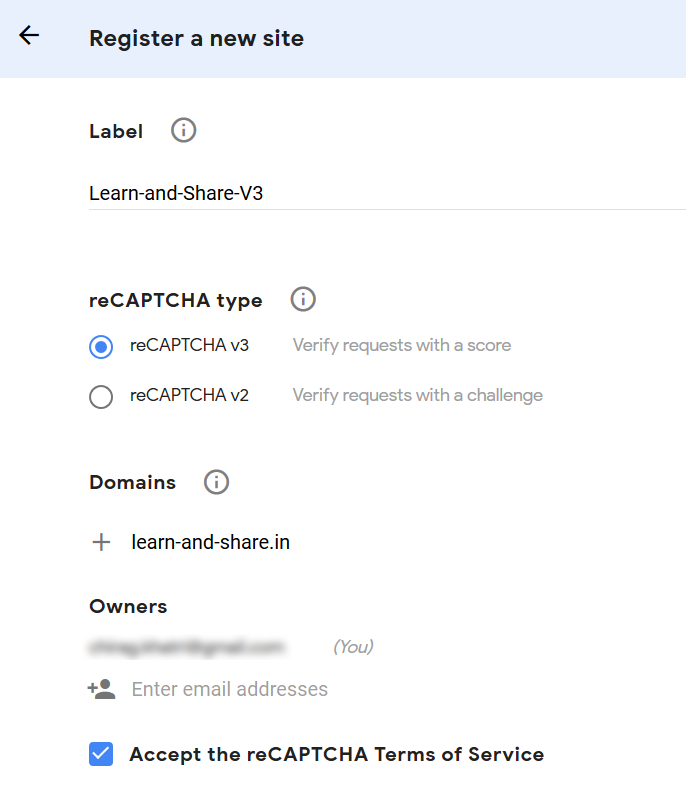
Fill the details properly and hit the “Submit” button. Google will generate the API Keys for you and display it on screen as below.
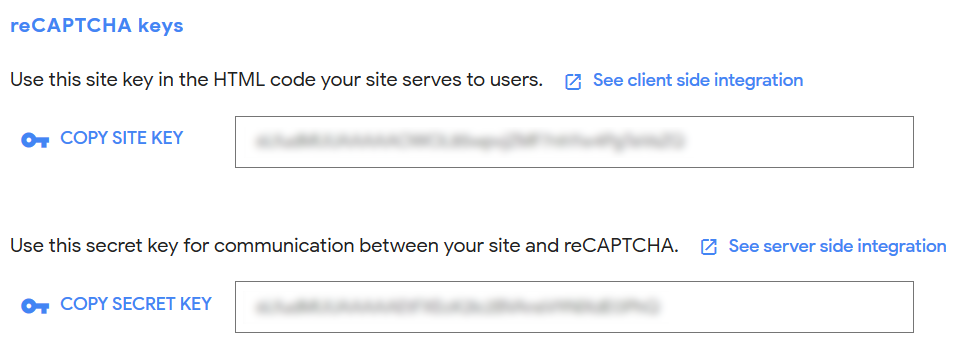
Now, click on the “See client-side integration” link to get the code snippet to put on your contact us form. The code looks like below:
<script><br />
grecaptcha.ready(function() {
grecaptcha.execute('_reCAPTCHA_site_key_', {action: 'homepage'}).then(function(token) {
...
});
});
</script>
- Load the JavaScript API with your site key
- Call grecaptcha.execute on an action or when the page loads
- Send the token to your backend with the request to verify
So your HTML Page looks like below after adding the Google reCaptcha code
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Contact Us</title>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<!------ Include the above in your HEAD tag ---------->
<style>
body {
background: -webkit-linear-gradient(left, #0072ff, #00c6ff);
}
.contact-form {
background: #fff;
margin-top: 5%;
margin-bottom: 5%;
width: 70%;
}
.contact-form .form-control {
border-radius:1rem;
}
.contact-image {
text-align: center;
}
.contact-image img {
border-radius: 6rem;
width: 11%;
margin-top: -3%;
transform: rotate(29deg);
}
.contact-form form {
padding: 14%;
}
.contact-form form .row {
}
.contact-form h3 {
margin-bottom: 8%;
margin-top: -10%;
text-align: center;
color: #0062cc;
}
.contact-form .btnContact {
width: 50%;
border: none;
border-radius: 1rem;
padding: 1.5%;
background: #dc3545;
font-weight: 600;
color: #fff;
cursor: pointer;
}
.btnContactSubmit {
width: 50%;
border-radius: 1rem;
padding: 1.5%;
color: #fff;
background-color: #0062cc;
border: none;
cursor: pointer;
}
</style>
<script src="https://www.google.com/recaptcha/api.js?render=__YOUR_SITE_KEY__"></script>
<?php
if(isset($_POST) && isset($_POST["btnSubmit"]))
{
$secretKey = 'YOUR_SECRET_KEY';
$token = $_POST["g-token"];
$ip = $_SERVER['REMOTE_ADDR'];
/* ======================= POST METHOD =====================*/
$url = "https://www.google.com/recaptcha/api/siteverify?";
$data = array('secret' => $secretKey, 'response' => $token, 'remoteip'=> $ip);
// use key 'http' even if you send the request to https://...
$options = array('http' => array(
'method' => 'POST',
'content' => http_build_query($data)
));
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
$response = json_decode($result);
if($response->success)
{
echo '<center><h1>Validation Success!</h1></center>';
}
else
{
echo '<center><h1>Captcha Validation Failed..!</h1></center>';
}
/*==========================================================*/
}
?>
</head>
<body>
<div class="container contact-form">
<form method="post">
<input type="hidden" id="g-token" name="g-token" />
<h3>Have a Question? Write us</h3>
<div class="row">
<div class="col-md-6">
<div class="form-group">
<input type="text" name="txtName" class="form-control" placeholder="Your Name *" value="" required />
</div>
<div class="form-group">
<input type="text" name="txtEmail" class="form-control" placeholder="Your Email *" value="" required />
</div>
<div class="form-group">
<input type="text" name="txtSubject" class="form-control" placeholder="Subject *" value="" required />
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<textarea name="txtMsg" class="form-control" placeholder="Your Message *" style="width: 100%; height:140px;" required></textarea>
</div>
</div>
</div>
<div class="row">
<div class="col-12">
<div class="form-group">
<input type="submit" name="btnSubmit" class="btnContact" value="Send Message" required />
</div>
</div>
</div>
</form>
</div>
<script>
grecaptcha.ready(function() {
grecaptcha.execute('__YOUR_SITE_KEY__', {action: 'homepage'}).then(function(token) {
console.log(token);
document.getElementById("g-token").value = token;
});
});
</script>
</body>
</html>
Your form like the below image. You can see the Google reCaptcha icon to the bottom right side of the page.
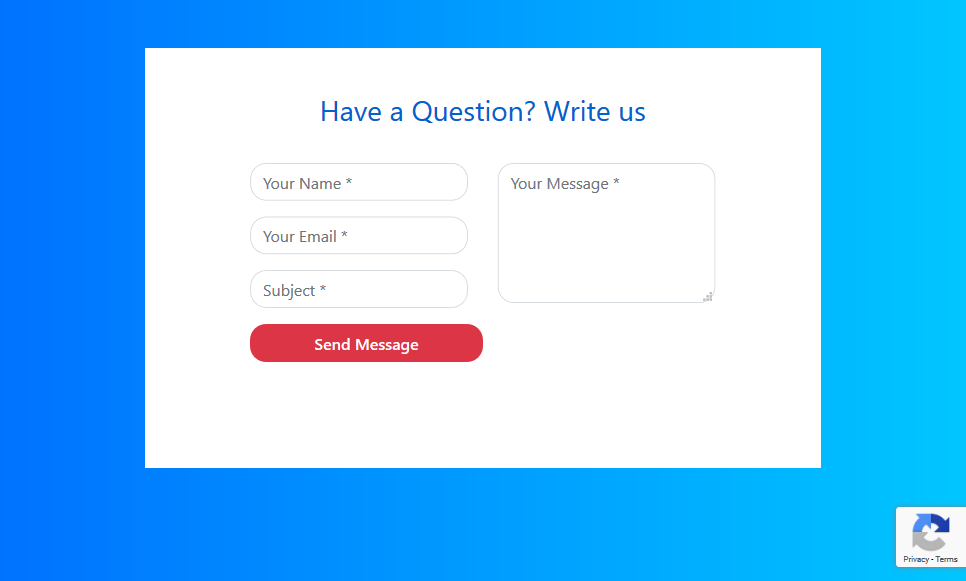
Chirag, the problem was my test server, works fine on production server; apparently have an issue in my openSSL implementation. Thanks again, you rock!
Hi Carlo
Thanks for the updates and figure out the issue. As a programmer, I can also suggest one thing to keep the habit to print the response of the API when you face any issue. It’ll give you more ideas about the bug and helping to find the solutions. In the tutorial, you can use the PHP function print_r to print the response getting from the server.
*Example:* print_r($captcha_response)
Thanks,
Chirag
Hi man, thank you very much for the tutorial, it has been really helpful for me to understand how to implement this feature in my website.
If you don’t mind I want to ask for your help because I’ve tried to implement the code using my localhost but I’m using TWO files instead of one and I’m having troubles get the response for the API.
The first file is the form itself in which I’ve added in the header:
Then a hidden tag inside my form:
And the script file at the bottom of the page:
grecaptcha.ready(function() {
grecaptcha.execute(‘_MY_SITE_KEY_’, {action: ‘homepage’}).then(function(token) {
console.log(token);
document.getElementById(“hdn_recaptcha_token”).value = token;
});
});
And the second file have server side validations, but before make those local DB validations I’ve added your code:
if(isset($_POST) && isset($_POST[‘hdn_recaptcha_token’])) {
$captcha_secret_key = “MY_SECRET_KEY_STRING”;
$captcha_client_key = $_POST[‘hdn_recaptcha_token’];
$captcha_client_ip = $_SERVER[‘REMOTE_ADDR’];
$captcha_url = “https://www.google.com/recaptcha/api/siteverify?”;
$captcha_data = array(
‘secret’ => $captcha_secret_key,
‘response’ => $captcha_client_key,
‘remoteip’=> $captcha_client_ip
);
$captcha_options = array(
‘http’ => array(
‘method’ => ‘POST’,
‘content’ => http_build_query($captcha_data)
)
);
$captcha_context = stream_context_create($captcha_options);
$captcha_result = file_get_contents($captcha_url, false, $captcha_context);
$captcha_response = json_decode($captcha_result);
if($captcha_response -> success)
{
echo ‘Validation Success!’;
} else {
echo ‘Captcha Validation Failed..!’;
}
}
But apparently I’m not getting the response from API google, so, I was wondering if you can see where’s the error in the code.
Thanks in advance,
–Carlo